Optional Chaining in ES7
What is Optional Chaining? Can we use optional chaining in React JS or in any JavaScript project?
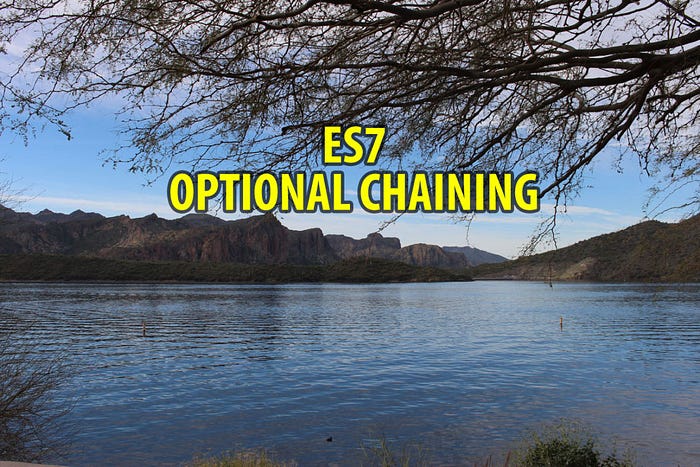
What is optional chaining?
I will try to explain it with an example.
I have an object, the value I have have is deep inside the nested object.

I want to check the value of dataExpiryDays and do something.
We can do this like this
if(data.user.feed.edgeReels.tray.node.dataExpiryDays) {
// do something
}
Wait…. anyone using JavaScript know the potential dangers of this approach. If any of the nested object is not available or null the above line of code will break.
Example if the key edgeReels is not available, you will get an error like Cannot access tray of undefined .
So we write the above code like this.
if ( data &&
data.user &&
data.user.feed &&
data.user.feed.edgeReels &&
data.user.feed.edgeReels.tray &&
data.user.feed.edgeReels.tray.node &&
data.user.feed.edgeReels.tray.node.dataExpiryDays ) {
// do something
}
See you need to do a check for every keys in each nested level.
To avoid these kind of checks, there was new feature proposal called Optional Chaining to be included in ES7. The proposal is now in Stage 4 (5/31/2020 — when I was writing this.) . See the proposal here.
The Optional Chaining operator
?.
With optional chaining operator we can write the above if condition like this.
if(data?.user?.feed?.edgeReels?.tray?.node?.dataExpiryDays) {
// do something
}
If any of the keys are missing subsequent checks are not done and the if condition fails.
Question 1: Can we validate an array position using Optional chaining?
Yes. like this
if(data?.nameArray?.[0]) {
// nameArray has index 0
}
Now the big questions.
- Can we use Optional chaining in my JavaScript project?
- Can we use Optional chaining in my React App?
Can we use Optional chaining in my JavaScript project?
No. As Optional chaining is still in proposal stage, most browsers will NOT have full support implemented. So I recommend not to use it unless you add polyfills.
Can we use Optional chaining in my React App?
React CRA uses Babel. Babel is a JavaScript transcompiler which makes new/next generation JavaScript features backward compatible with all browsers(don’t expect IE older versions).
So if you use React or Gastby or any other JavaScript project uses Babel 7.8 or above versions you are good to use Optional Chaining. If you are using React CRA check Babel version inside node-modules/@babel/core/package.json or check for a folder plugin-proposal-optional-chaining inside node-modules/@babel/
So check it out and post your comments if any.
Happy coding…. 🍺
Originally published at https://kiranvj.com on May 31, 2020.